Transform your Raspberry Pi into a powerful time-lapse photography hub by mastering the core components: a quality camera module, reliable power supply, and Python scripting knowledge. After grasping the Raspberry Pi basics, time-lapse photography opens up endless creative possibilities, from capturing stunning natural phenomena to documenting long-term projects with precision.
Modern Raspberry Pi cameras now capture up to 12.3 megapixels with remarkable low-light performance, making them ideal for both indoor and outdoor time-lapse projects. Combined with the Pi’s processing power, you can automatically adjust exposure settings, implement motion detection, and even process images in real-time – capabilities that traditionally required expensive professional equipment.
Whether you’re documenting plant growth, construction progress, or cloud formations, the Raspberry Pi’s compact size, energy efficiency, and robust software ecosystem make it the perfect platform for time-lapse photography. With the right setup, your Pi can run autonomously for weeks or months, capturing and processing thousands of images while maintaining consistent quality and timing.
Essential Hardware Setup
Camera Selection and Mounting
The Raspberry Pi Camera Module v2 and the newer HQ Camera Module are ideal choices for time-lapse projects, offering excellent image quality and direct compatibility with the Pi. The v2 module, with its 8MP sensor, provides a good balance of quality and affordability, while the 12.3MP HQ Camera delivers superior results for more demanding projects.
When mounting your camera, stability is crucial for consistent time-lapse results. Consider using a sturdy tripod mount or creating a custom mounting bracket using 3D-printed parts. The camera should be firmly secured to prevent any movement during the capture period, as even slight shifts can ruin your time-lapse sequence.
For outdoor installations, protect your setup from the elements using a weatherproof case. Position the camera where it won’t be affected by direct sunlight or rain, and ensure proper ventilation to prevent condensation on the lens. The camera cable should be properly secured and protected, with no sharp bends that could damage the connection over time.
If you’re capturing long-term sequences, consider mounting options that allow for easy access to the Pi for maintenance while keeping the camera position fixed.
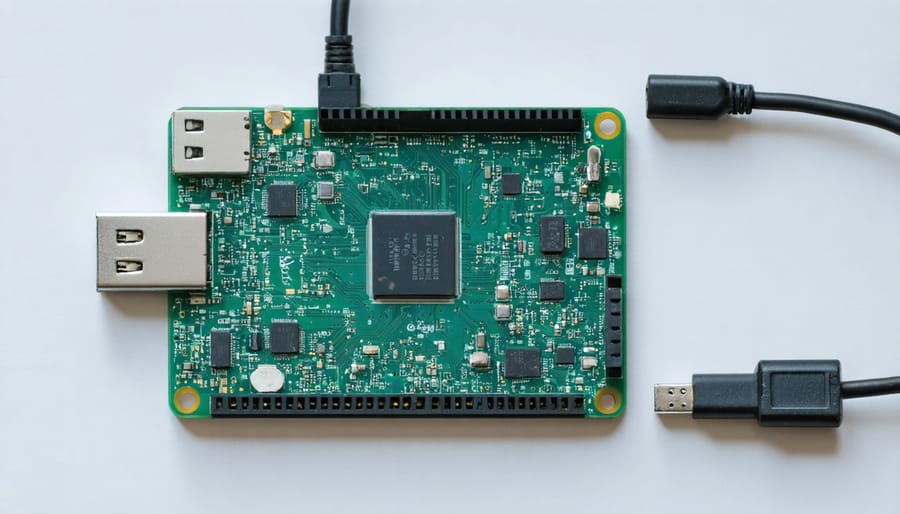
Power Management Solutions
Reliable power management is crucial for successful long-term time-lapse projects with your Raspberry Pi. The most straightforward solution is connecting to mains power through a high-quality 5V power supply rated at 2.5A or higher. However, for outdoor or remote locations, you’ll need alternative power solutions.
A popular option is using a UPS (Uninterruptible Power Supply) HAT, which provides backup power during main power interruptions. These HATs typically include a battery pack and intelligent power management features, ensuring your Pi shuts down safely if power runs low.
For completely off-grid setups, consider combining a solar panel with a large-capacity power bank. A 20W solar panel paired with a 20000mAh power bank can keep your Pi running for several days. Remember to include a voltage regulator to protect your Pi from power fluctuations.
To maximize battery life, implement power-saving techniques like disabling unused USB ports, turning off HDMI output, and scheduling your Pi to sleep between shots. These optimizations can extend your battery life by up to 30%.
Software Implementation
Installing Required Libraries
Before we begin capturing time-lapse footage, we need to install several essential Python libraries on your Raspberry Pi. Open your terminal and enter the following commands:
“`bash
sudo apt-get update
sudo apt-get upgrade
sudo apt-get install python3-pip
“`
Next, install the required libraries for image capture and processing:
“`bash
pip3 install picamera
pip3 install opencv-python
pip3 install pillow
“`
The picamera library enables control of the Raspberry Pi camera module, while opencv-python provides powerful image processing capabilities. Pillow (PIL) handles image file operations and basic manipulations.
If you plan to create video outputs from your time-lapse images, you’ll also need FFmpeg:
“`bash
sudo apt-get install ffmpeg
“`
Some users might encounter memory errors while installing OpenCV. If this happens, try using the lightweight version instead:
“`bash
pip3 install opencv-python-headless
“`
After installation, verify everything is working correctly by running Python and importing the libraries:
“`python
import picamera
import cv2
from PIL import Image
“`
If no errors appear, you’re ready to start capturing your time-lapse sequences. Remember to reboot your Raspberry Pi if you experience any unusual behavior after installation.
Writing the Time-Lapse Script
Let’s create a Python script that will handle our time-lapse photography. Create a new file called timelapse.py and input the following code:
“`python
from picamera import PiCamera
from time import sleep
from datetime import datetime
import os
# Initialize camera
camera = PiCamera()
camera.resolution = (1920, 1080) # Full HD resolution
camera.rotation = 0 # Adjust if needed (0, 90, 180, or 270)
# Customizable parameters
INTERVAL = 60 # Time between shots in seconds
DURATION = 24 # Duration in hours
FOLDER = ‘timelapse_images’ # Storage folder
# Create storage directory
if not os.path.exists(FOLDER):
os.makedirs(FOLDER)
# Calculate total shots
total_shots = (DURATION * 3600) // INTERVAL
print(f”Starting time-lapse: {total_shots} images over {DURATION} hours”)
for i in range(total_shots):
timestamp = datetime.now().strftime(“%Y%m%d_%H%M%S”)
filename = f”{FOLDER}/image_{timestamp}.jpg”
camera.start_preview()
sleep(2) # Camera warm-up
camera.capture(filename)
camera.stop_preview()
print(f”Captured {i+1}/{total_shots}: {filename}”)
sleep(INTERVAL – 2) # Subtract warm-up time
“`
You can easily customize the script by adjusting the parameters at the top. INTERVAL controls how often photos are taken, DURATION sets how long the time-lapse will run, and FOLDER specifies where images are stored. The script automatically creates timestamped filenames and displays progress as it runs.
For longer time-lapses, consider adding error handling and automatic cleanup of old images to manage storage space. The script can be started automatically at boot by adding it to your crontab or running it as a system service.
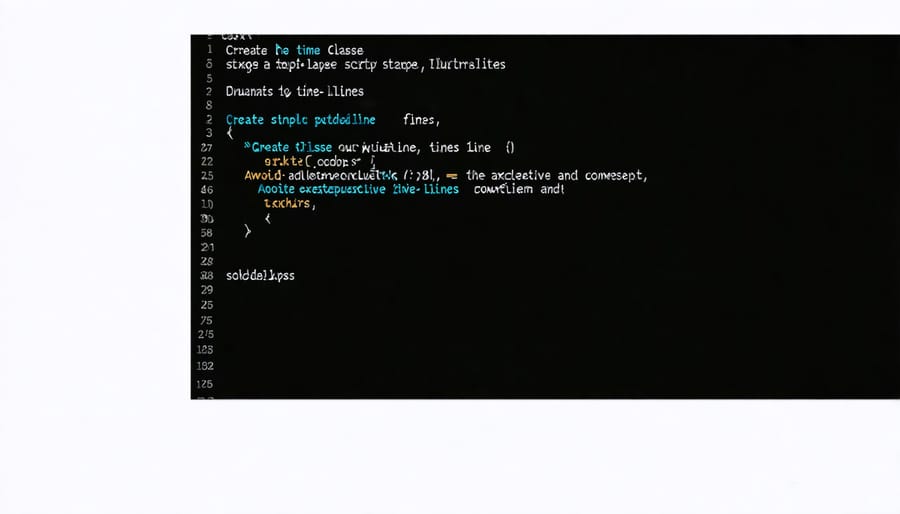
Automating Capture Sessions
To automate your time-lapse capture sessions, the Raspberry Pi’s built-in cron scheduler is your best friend. Start by opening the terminal and typing ‘crontab -e’ to edit your cron jobs. For a basic daily capture schedule, add a line like:
0 8 * * * python /home/pi/timelapse/capture.py
This command runs your capture script every day at 8 AM. For more frequent captures, you can adjust the timing using the standard cron format. For example, to capture an image every 5 minutes:
*/5 * * * * python /home/pi/timelapse/capture.py
Create a simple Python script (capture.py) that handles the image capture:
“`python
from picamera import PiCamera
from datetime import datetime
import time
camera = PiCamera()
timestamp = datetime.now().strftime(‘%Y%m%d_%H%M%S’)
camera.capture(f’/home/pi/timelapse/image_{timestamp}.jpg’)
“`
Remember to test your automation script manually before setting up the cron job. Also, consider adding error handling and logging to monitor your capture sessions and ensure everything runs smoothly over extended periods.
Real-Time Processing Features
Image Processing Options
Image processing plays a crucial role in creating stunning time-lapse sequences with your Raspberry Pi. By leveraging the Pi’s real-time processing capabilities, you can implement various adjustments and filters to enhance your footage as it’s being captured.
Start with basic exposure controls to maintain consistent lighting throughout your sequence. The raspistill command supports parameters like –ev (exposure compensation) and –awb (auto white balance) to fine-tune your image quality. For example, setting –ev 2 increases exposure by two stops, useful for low-light scenarios.
Consider implementing automatic brightness correction to handle changing lighting conditions. This can be achieved using Python libraries like PIL (Python Imaging Library) or OpenCV. These tools allow you to analyze histogram data and adjust image parameters on the fly.
For more advanced processing, explore options like:
– Contrast enhancement using adaptive histogram equalization
– Color temperature adjustment to maintain consistency
– Noise reduction filters for cleaner images
– Sharpening filters to enhance detail
– HDR merging for high-contrast scenes
Remember to balance processing complexity with your Pi’s resources. Heavy image processing can impact capture timing and potentially create gaps in your sequence. Start with essential adjustments and gradually add more sophisticated filters as needed.
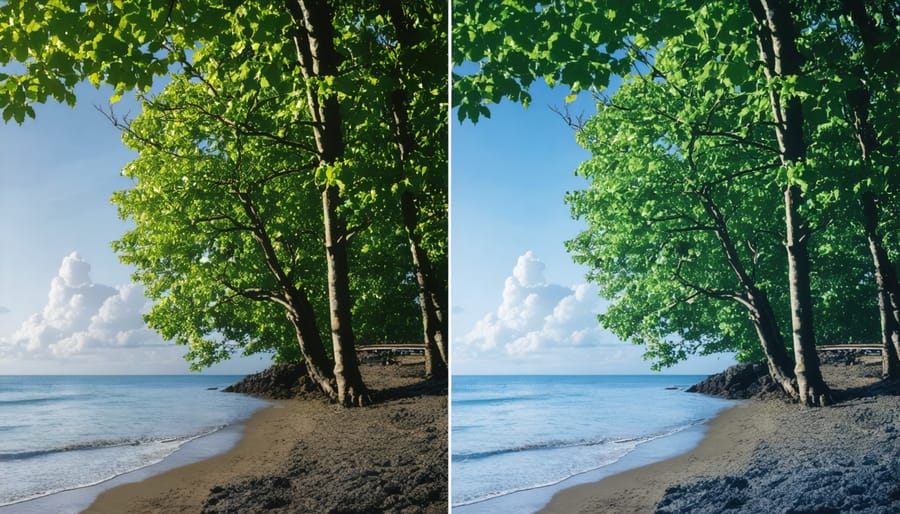
Performance Optimization
Running a time-lapse capture over extended periods requires careful attention to system resources and storage management. To optimize performance, start by implementing a cleanup routine that automatically removes temporary files after processing. Consider using a RAM disk for temporary storage of images before they’re written to the main storage, which reduces wear on your SD card and improves capture speed.
Monitor your system’s temperature, especially during daylight hours when both sunlight and processing can cause heating issues. Installing a small cooling fan or heat sinks can prevent thermal throttling and maintain consistent capture intervals. Set up automatic email alerts for when system temperature exceeds safe thresholds.
Storage management is crucial for long sessions. Implement a rolling deletion system that removes older processed files while maintaining your raw captures. Use efficient image compression settings that balance quality with file size. Consider connecting an external USB drive for additional storage capacity.
Enable logging to track system performance and capture success rates. This helps identify potential issues before they become critical. Schedule periodic system reboots during natural breaks in your time-lapse sequence to clear memory and prevent potential system hangups. These practices ensure smooth operation throughout your entire capture period.
Troubleshooting and Best Practices
Common Technical Challenges
When setting up a time-lapse project with your Raspberry Pi, you might encounter several common technical challenges. Here’s how to address them effectively:
Storage space issues often arise during extended shoots. Monitor your free space regularly and consider using external storage devices or implementing automatic file cleanup scripts to prevent your SD card from filling up.
Camera module connection errors are another frequent hurdle. If you see “Camera not detected” or “MMAL” errors, double-check your ribbon cable connection and ensure it’s properly seated in both the camera and Pi board. Sometimes, simply running “raspi-config” and enabling the camera interface resolves these issues.
Power interruptions can corrupt your project. Use a reliable power supply and consider adding a UPS (Uninterruptible Power Supply) for outdoor or long-term setups. Adding a safe shutdown script can help protect your data if power issues occur.
Temperature-related problems may affect outdoor installations. If your Pi shows the thermometer icon or throttles performance, install it in a well-ventilated case and avoid direct sunlight exposure.
Network connectivity drops can disrupt remote monitoring. Set up a local backup solution and implement automatic reconnection scripts. For Wi-Fi installations, consider using a high-gain antenna or positioning your Pi closer to the router.
Image quality issues like focus drift or exposure variations can be prevented by locking your camera settings and using manual mode where possible. Test your setup thoroughly before starting a long-term capture sequence.
Photography Tips
To capture stunning time-lapse sequences with your Raspberry Pi setup, follow these professional photography tips that will elevate your results. First, ensure your camera is completely stable by using a solid mounting solution or tripod. Even minor vibrations can create noticeable shakiness in your final video.
Consider your frame composition carefully before starting. The rule of thirds remains important in time-lapse photography – position key elements along these invisible grid lines for more engaging shots. When capturing nature or outdoor scenes, include both static and moving elements to create visual interest.
Lighting changes are crucial in time-lapse photography. For outdoor shoots, plan your timing around the “golden hours” (just after sunrise or before sunset) for the most dramatic lighting effects. For indoor sequences, maintain consistent lighting throughout the capture period to avoid flickering in your final video.
Set your camera’s exposure settings manually to prevent automatic adjustments between frames, which can cause unwanted flickering. Start with these baseline settings: ISO 100-400 for daylight, shutter speed 1/50-1/100, and aperture f/8-f/11 for good depth of field.
Choose your interval timing based on your subject. Use shorter intervals (1-5 seconds) for fast-moving subjects like clouds or traffic, and longer intervals (30-60 seconds) for slower changes like plant growth or construction projects. Remember, longer projects require careful power management and storage planning.
Time-lapse photography with Raspberry Pi opens up endless creative possibilities for both beginners and experienced makers. By following the steps and tips outlined in this guide, you can create stunning time-lapse videos that capture everything from blooming flowers to bustling cityscapes. The flexibility and affordability of Raspberry Pi make it an ideal platform for these projects, joining the growing list of innovative Raspberry Pi applications in photography and beyond. Whether you’re documenting construction projects, tracking plant growth, or creating artistic sequences, the skills you’ve learned here will serve as a foundation for more advanced projects. Don’t be afraid to experiment with different settings, intervals, and subjects – the best way to master time-lapse photography is through hands-on experience. Start your time-lapse journey today and share your creative vision with the world.