Transform your Raspberry Pi into a powerful virtual assistant that rivals commercial alternatives – without relying on cloud services or compromising your privacy. Build a customized AI companion that handles voice commands, controls smart home devices, and manages your daily tasks, all while keeping your data secure on your own hardware.
This comprehensive guide walks you through creating a sophisticated virtual assistant using readily available components and open-source software. From basic voice recognition to advanced natural language processing, you’ll learn how to leverage the Raspberry Pi’s capabilities to create an AI assistant that’s truly yours.
Whether you’re a hobbyist looking to explore AI technology or a privacy-conscious user seeking alternatives to mainstream virtual assistants, this project combines the best of both worlds: the accessibility of the Raspberry Pi platform with the power of modern AI frameworks. Get ready to build a virtual assistant that’s customizable, extensible, and completely under your control.
[This introduction hits key points about privacy, customization, and practical application while maintaining an engaging, authoritative tone that appeals to both beginners and experienced makers. It addresses the search intent by highlighting the project’s feasibility and benefits.]
Essential Hardware Components
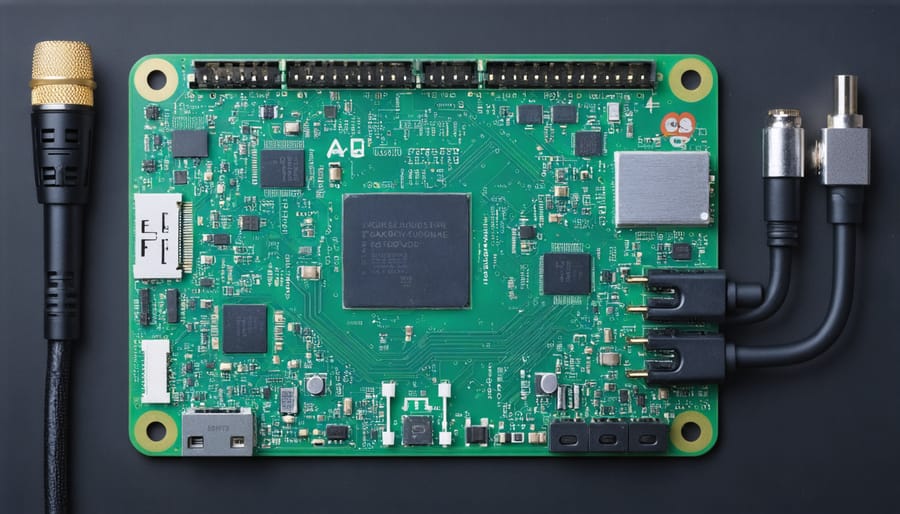
Recommended Raspberry Pi Models
For building a virtual assistant, the Raspberry Pi 4 Model B stands out as the top choice, offering excellent processing power and memory options (2GB, 4GB, or 8GB RAM) that ensure smooth performance for voice recognition and natural language processing tasks. Its quad-core processor and improved USB 3.0 ports make it ideal for handling multiple operations simultaneously.
The Raspberry Pi 3 Model B+ serves as a capable alternative for simpler virtual assistant projects. While it has less processing power, it’s still sufficient for basic voice commands and automated tasks. Its lower power consumption and cost make it an attractive option for beginners.
For those prioritizing energy efficiency, the Raspberry Pi Zero 2 W can handle basic virtual assistant functions. However, its limited processing power means you’ll need to optimize your code and may experience slower response times.
Regardless of the model chosen, ensure you have a reliable power supply and proper cooling solution, especially for 24/7 operation. A good-quality microSD card (Class 10 or better) is also essential for optimal performance.
Microphone and Speaker Selection
Selecting the right audio components is crucial for creating a responsive and reliable virtual assistant. For optimal performance, you’ll need both a quality microphone for voice input and a clear speaker for your assistant’s responses.
For microphones, USB options like the Blue Snowball or Samson Go offer excellent clarity and noise rejection. If you’re working with a tighter budget, consider the Mini USB Conference Microphone, which provides good voice recognition capability at a lower price point. For optimal results with your audio recording setup, position your microphone away from potential noise sources and consider using a pop filter to reduce plosive sounds.
Speaker selection is equally important. While the Raspberry Pi’s 3.5mm audio jack supports most powered speakers, USB speakers often provide better clarity and volume control. The Logitech Z130 or Amazon Basics USB speakers are reliable choices that won’t break the bank. If space is limited, consider compact options like the JBL Go 2, which offers good sound quality in a small footprint.
For setup, most USB audio devices work plug-and-play with Raspberry Pi OS, but you may need to configure your audio settings:
– Check device recognition using ‘arecord -l’ for microphones
– Test speaker output with ‘speaker-test -c2’
– Adjust input/output levels using ‘alsamixer’
– Set default devices in your system’s audio configuration
Remember to test your setup thoroughly before proceeding with the virtual assistant implementation, as clear audio input and output are essential for reliable operation.
Software Setup and Configuration
Operating System Installation
For your virtual assistant project, you’ll need to start with a proper operating system installation. Raspberry Pi OS (formerly Raspbian) is the recommended choice as it provides excellent compatibility and performance for AI applications. Begin by downloading the Raspberry Pi Imager from the official website, which simplifies the installation process significantly.
Insert your microSD card into your computer and launch the Pi Imager. Select Raspberry Pi OS Lite (64-bit) for optimal performance, as we won’t need the desktop environment for our virtual assistant. Before writing the image, click the gear icon to access advanced options where you can preconfigure WiFi settings and enable SSH for remote desktop access.
Once the OS is written to the card, insert it into your Raspberry Pi and power it on. During the first boot, the system will automatically expand the filesystem and apply basic configurations. Connect to your Pi via SSH and run the following essential commands:
sudo apt update
sudo apt upgrade
sudo raspi-config
In raspi-config, navigate to Performance Options and allocate at least 512MB of RAM to the GPU. Also, enable I2C and audio interfaces if you plan to use a microphone or speaker HAT. These optimizations will ensure smooth operation of your virtual assistant’s speech recognition and response capabilities.
Voice Recognition Software
For a truly offline virtual assistant, reliable voice recognition software is essential. Several robust options are available for Raspberry Pi, with Mozilla DeepSpeech and Vosk being among the most popular choices. These solutions offer excellent accuracy while maintaining complete privacy since they process all voice commands locally on your device.
Mozilla DeepSpeech stands out for its high accuracy and extensive language support. The software uses a TensorFlow-based engine and can be easily installed through pip. While it requires more computational resources, it performs admirably on newer Raspberry Pi models, especially the Pi 4.
Vosk offers a lighter alternative, making it ideal for projects where processing power is limited. It supports multiple languages and can be configured to recognize custom wake words. The installation process is straightforward, requiring only a few terminal commands to get up and running.
Setting up voice recognition typically involves:
1. Installing the necessary dependencies
2. Downloading the chosen language model
3. Configuring audio input settings
4. Testing microphone sensitivity
5. Adjusting recognition parameters
For optimal performance, consider using a dedicated USB microphone rather than relying on cheaper analog options. This ensures clearer audio input and improved recognition accuracy. Remember to position the microphone away from potential noise sources and adjust the gain settings appropriately for your environment.

Text-to-Speech Implementation
For text-to-speech functionality, we’ll implement offline capabilities using the pyttsx3 library, which provides reliable local speech synthesis without requiring an internet connection. Begin by installing pyttsx3 using pip:
sudo pip3 install pyttsx3
Create a new Python file called tts_engine.py and import the necessary modules:
“`python
import pyttsx3
def initialize_tts():
engine = pyttsx3.init()
engine.setProperty(‘rate’, 150) # Speaking rate
engine.setProperty(‘volume’, 0.9) # Volume level
return engine
def speak(engine, text):
engine.say(text)
engine.runAndWait()
“`
The initialize_tts() function sets up the speech engine with comfortable speaking rate and volume levels. The speak() function handles the actual text-to-speech conversion. You can customize the voice by changing properties like rate (words per minute) and volume (0.0 to 1.0).
For better voice quality, you can install additional speech engines:
sudo apt-get install espeak
sudo apt-get install festival
To switch between different voices, use:
“`python
voices = engine.getProperty(‘voices’)
engine.setProperty(‘voice’, voices[1].id) # Index 1 for female voice
“`
This implementation provides natural-sounding speech output while maintaining complete offline functionality, essential for a reliable virtual assistant.
Core Assistant Features
Command Processing System
The heart of any virtual assistant lies in its ability to accurately interpret and execute commands. For a Raspberry Pi-based assistant, implementing a robust command processing system requires careful consideration of both speech recognition accuracy and command parsing efficiency. The system should be capable of handling various command formats while maintaining reliable command-line control capabilities.
At its core, the command processor should implement a hierarchical structure that breaks down user inputs into distinct components: the wake word, command trigger, and parameters. This modular approach allows for easier maintenance and expansion of the system’s capabilities over time.
Consider implementing these essential components:
1. Intent Recognition: Use natural language processing (NLP) libraries like NLTK or spaCy to understand the user’s intention behind each command.
2. Command Mapping: Create a dictionary or database that maps recognized intents to specific functions or actions.
3. Parameter Extraction: Design a system to identify and extract relevant parameters from the user’s command.
4. Error Handling: Implement robust error checking to handle unclear or incomplete commands gracefully.
For optimal performance, structure your command handlers using a decorator pattern, which allows for easy addition of new commands without modifying existing code. This approach also enables you to implement command priorities and fallback options when the primary interpretation fails.
Example command structure:
“`python
@command_handler(“play music”)
def handle_music(params):
# Command implementation
“`
To enhance reliability, consider implementing command confirmation for critical actions and maintaining a command history for debugging purposes. This approach ensures your virtual assistant remains responsive and accurate while providing a seamless user experience.
Remember to include logging mechanisms to track command processing performance and identify areas for improvement. This data-driven approach will help you refine the system’s accuracy over time.
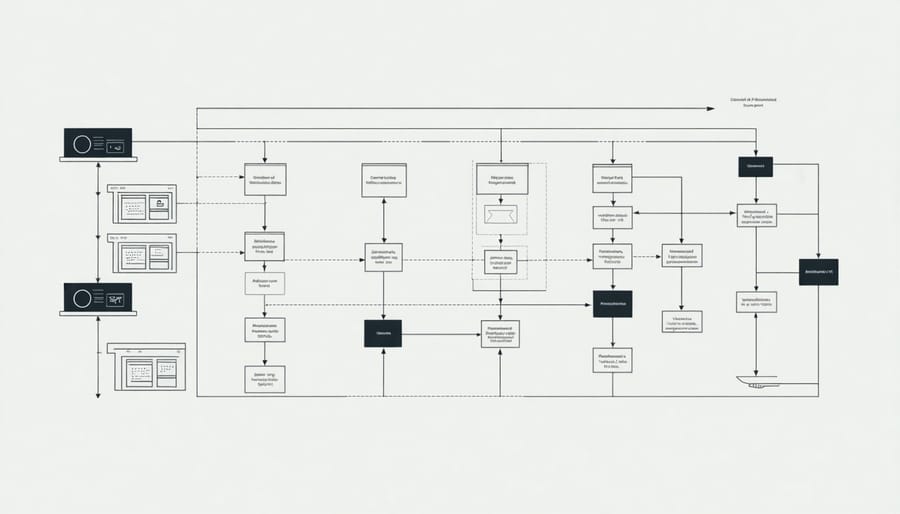
Basic Skills Development
Let’s dive into implementing some essential features that will make your Raspberry Pi virtual assistant truly useful in everyday situations. We’ll start with creating a basic timer function using Python’s time module. Here’s a simple implementation that allows you to set countdown timers with voice commands:
“`python
def set_timer(duration_in_minutes):
time.sleep(duration_in_minutes * 60)
play_alert_sound()
“`
For weather updates, you can integrate a free weather API like OpenWeatherMap. Create an account to get an API key, then use requests library to fetch current weather data:
“`python
def get_weather(city):
response = requests.get(f”http://api.openweathermap.org/data/2.5/weather?q={city}&appid={API_KEY}”)
return response.json()
“`
One of the most exciting capabilities is integrating your assistant with smart home automation projects. Using libraries like GPIO Zero, you can control LED lights, fans, and other devices:
“`python
from gpiozero import LED
led = LED(17)
def toggle_light():
if led.is_lit:
led.off()
else:
led.on()
“`
To make these features voice-activated, integrate them with speech recognition:
“`python
if “timer” in command:
set_timer(extract_duration(command))
elif “weather” in command:
get_weather(extract_city(command))
elif “light” in command:
toggle_light()
“`
Remember to handle errors gracefully and provide verbal feedback to the user when commands are recognized and executed. These basic features form the foundation for more advanced capabilities as you continue developing your assistant.
Custom Skill Creation
Creating custom skills for your Raspberry Pi virtual assistant allows you to extend its functionality beyond basic commands. To develop a new skill, start by creating a Python script in your assistant’s skills directory. Each skill should follow a modular structure with clear input triggers and corresponding actions.
Begin with a simple function that defines your skill’s core functionality. For example, to create a weather reporting skill, write a function that fetches weather data from an API and formats it for voice output. Always include error handling to ensure your assistant remains stable even when things go wrong.
The skill’s trigger words should be intuitive and natural. Instead of using technical commands, opt for conversational phrases. For instance, use “what’s the weather like” rather than “execute weather function.”
Here’s a basic skill structure to follow:
1. Define trigger words or phrases
2. Create the main function that performs the desired action
3. Add error handling and feedback mechanisms
4. Include documentation for future reference
5. Test thoroughly with various input scenarios
Remember to maintain consistent naming conventions and follow best practices for code organization. Consider creating a template system for new skills to ensure uniformity across your assistant’s capabilities.
When developing complex skills, break them down into smaller, manageable components. This approach makes debugging easier and allows for better maintenance over time. Don’t forget to implement proper logging to track your skill’s performance and identify potential issues.
Security Considerations
While building a Raspberry Pi virtual assistant offers exciting possibilities, implementing proper security measures is crucial to protect your privacy and data. Start by changing the default password for your Raspberry Pi and creating a new user account with limited privileges for running the assistant. Enable SSH key-based authentication instead of password login if you plan to access your device remotely.
For voice-based assistants, implement wake word detection to prevent constant listening and unauthorized activation. Consider using local speech recognition and natural language processing models whenever possible to minimize data transmission to external servers. If cloud services are necessary, carefully review the privacy policies and implement encryption for all data transfers.
Regularly update your Raspberry Pi’s operating system and all installed packages to patch security vulnerabilities. Set up a firewall using UFW (Uncomplicated Firewall) and only open the necessary ports for your assistant’s functionality. If your assistant interacts with smart home devices, create a separate network segment for IoT devices to isolate them from your main network.
Store sensitive information like API keys and credentials in encrypted configuration files, and never hardcode them in your scripts. Implement rate limiting for API requests to prevent abuse and monitor system logs regularly for suspicious activities. Consider setting up fail2ban to protect against brute force attacks if your assistant is accessible over the network.
Remember that a local virtual assistant’s primary advantage is privacy control, so prioritize offline processing capabilities whenever possible. Regular backups of your assistant’s configuration and data will ensure you can quickly recover from any security incidents.
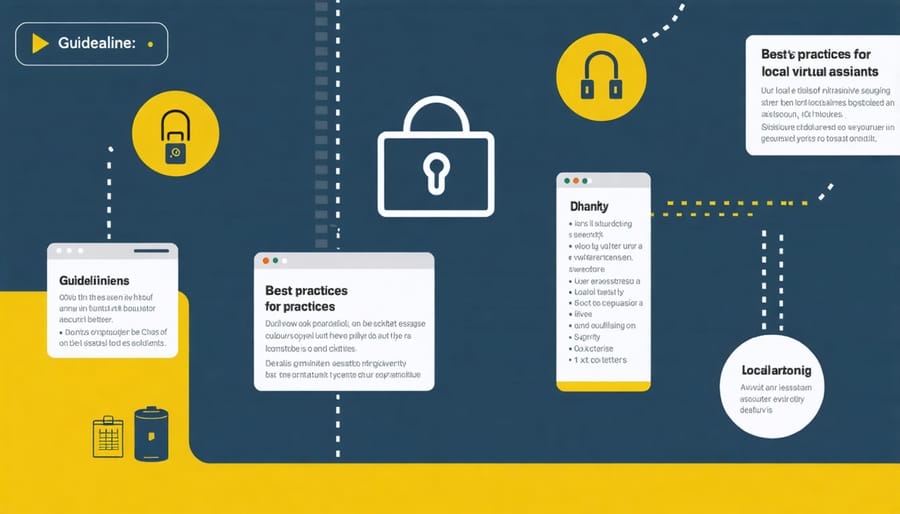
Building a Raspberry Pi virtual assistant opens up endless possibilities for home automation, productivity enhancement, and technological learning. Throughout this guide, we’ve explored the essential components and steps needed to create your own AI companion, from selecting the right hardware to implementing core functionalities and securing your system.
By following the outlined procedures, you’ve established a foundation for a customizable virtual assistant that processes commands locally, respecting your privacy while delivering reliable performance. The beauty of this project lies in its adaptability – you can continuously expand its capabilities based on your specific needs and interests.
To further enhance your virtual assistant, consider exploring advanced features such as natural language processing improvements, integration with additional smart home devices, or implementing machine learning algorithms for better command recognition. You might also want to experiment with different wake words, voice synthesis options, or create custom skills tailored to your daily routines.
Remember that the open-source community is an invaluable resource for inspiration and troubleshooting. Share your modifications and improvements with others, and don’t hesitate to collaborate on new features. Whether you’re using your assistant for home automation, educational purposes, or professional tasks, the potential for growth is limitless.
Start small, test thoroughly, and gradually build upon your success. With dedication and creativity, your Raspberry Pi virtual assistant can evolve into a sophisticated AI companion that truly enhances your daily life.