Transform your Raspberry Pi into a sophisticated battery management system (BMS) by combining precision voltage monitoring, real-time data logging, and intelligent charge control capabilities. This powerful combination enables DIY enthusiasts to create professional-grade power management solutions, similar to solar-powered Raspberry Pi systems. Harness the Pi’s GPIO pins to interface with voltage sensors, implement Python-based monitoring scripts, and develop custom web dashboards for remote battery status tracking. Whether managing a single battery or orchestrating complex multi-cell configurations, the Raspberry Pi’s versatility makes it an ideal platform for building cost-effective, customizable BMS solutions that rival commercial alternatives.
Understanding BMS Basics for Raspberry Pi
Essential Hardware Components
To build a reliable Battery Management System (BMS) with your Raspberry Pi, you’ll need several key hardware components. Understanding the Raspberry Pi power requirements is crucial before starting your build. The essential components include:
A voltage sensor module (like ADS1115 or INA219) for accurate battery voltage monitoring, current sensing capabilities for tracking charge/discharge rates, and a temperature sensor (such as DHT22 or DS18B20) to monitor battery temperature for safety.
You’ll also need:
– Battery connection terminals
– Level shifter (3.3V to 5V logic conversion)
– Protective circuitry (fuses and reverse polarity protection)
– GPIO expansion board (optional but recommended)
– LCD display or LED indicators for status monitoring
– High-quality wires and connectors
For reliable data collection, ensure all sensors are properly calibrated and connected to the appropriate GPIO pins. A breadboard and jumper wires are helpful for initial prototyping before moving to a more permanent solution. Consider adding a real-time clock module (RTC) if you need precise timing for data logging.
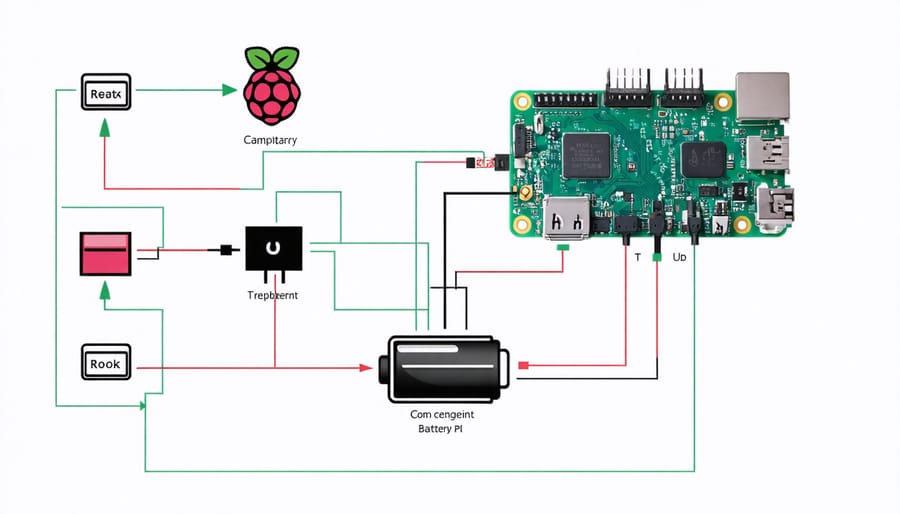
Software Requirements
To build a BMS system with a Raspberry Pi, you’ll need several key software components installed. Start by ensuring your Raspberry Pi runs the latest version of Raspberry Pi OS (formerly Raspbian), which provides the foundation for your BMS project.
Essential Python libraries include:
– RPi.GPIO for GPIO pin control
– SMBus2 for I2C communication
– NumPy for data calculations
– Matplotlib for real-time data visualization
– Flask for web interface development (if implementing remote monitoring)
Install these packages using pip:
“`
pip install RPi.GPIO smbus2 numpy matplotlib flask
“`
You’ll also need to enable I2C communication through raspi-config:
1. Access raspi-config via terminal
2. Navigate to Interface Options
3. Enable I2C interface
For data logging and storage, install SQLite:
“`
sudo apt-get install sqlite3
“`
Additional recommended tools include Git for version control and screen or tmux for managing multiple terminal sessions. Ensure your Python version is 3.7 or higher for compatibility with all required libraries.
Setting Up Your Raspberry Pi BMS Hardware
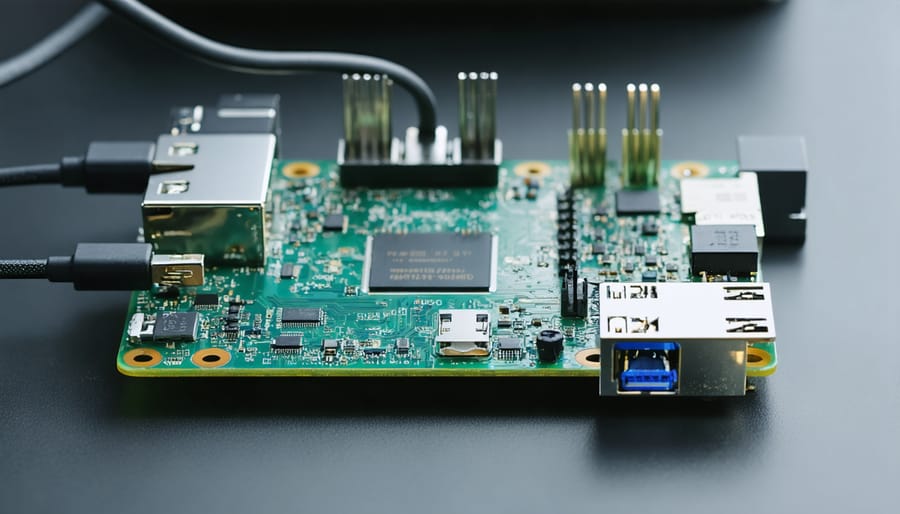
Wiring and Connections
To connect your BMS to a Raspberry Pi, you’ll need to establish proper wiring connections that ensure safe and reliable communication between components. Start by connecting the BMS’s UART/RS232 interface to the Raspberry Pi’s GPIO pins:
1. Connect the BMS TX pin to GPIO15 (RXD0, Pin 10)
2. Connect the BMS RX pin to GPIO14 (TXD0, Pin 8)
3. Connect the BMS GND to any Raspberry Pi GND pin
4. Connect the BMS VCC to the 3.3V pin if required
For voltage monitoring, wire the BMS sense leads to your battery pack:
– B- (Battery negative) to the pack’s negative terminal
– B1, B2, etc. to respective cell connection points
– B+ (Battery positive) to the pack’s positive terminal
When using additional sensors:
– Temperature sensors connect to GPIO4 (Pin 7)
– Current sensor output to GPIO17 (Pin 11)
– Status LED to GPIO18 (Pin 12)
Safety considerations:
– Use appropriate gauge wires for power connections
– Add inline fuses for protection
– Ensure all connections are properly insulated
– Double-check polarity before powering on
– Use heat shrink tubing on exposed connections
For enhanced reliability:
– Secure all connections with proper terminals or connectors
– Keep high-current wires separate from signal wires
– Use shielded cables for data connections
– Install pull-up/pull-down resistors where necessary
Test all connections with a multimeter before applying power to ensure everything is correctly wired and there are no short circuits.
Safety Considerations
When working with a battery management system (BMS) and Raspberry Pi combination, safety should be your top priority. Always handle lithium-ion batteries with extreme care, as improper usage can lead to dangerous situations. Ensure your workspace is well-ventilated and keep a fire extinguisher rated for electrical fires nearby.
Install your BMS setup in a properly insulated enclosure to prevent short circuits and protect against environmental factors. Monitor temperature readings consistently – excessive heat can damage both your batteries and the Raspberry Pi. Consider implementing an automatic shutdown feature if temperatures exceed safe thresholds.
Power management is crucial for an eco-friendly Raspberry Pi setup. Use appropriate fuses and circuit breakers to protect against overcurrent situations. Never connect batteries directly to the Raspberry Pi without proper voltage regulation and protection circuits in place.
Keep your wiring neat and organized, using appropriate gauge wires for your current requirements. Label all connections clearly and document your setup thoroughly. Avoid exposed wires and use heat-shrink tubing or electrical tape to insulate connections.
Regular maintenance checks are essential. Monitor for any signs of battery swelling, unusual odors, or physical damage. Keep your code updated with proper error handling to prevent system failures that could lead to safety issues.
When testing new features or modifications, always start with a low-voltage setup before scaling up. If you’re new to BMS projects, consider working with more experienced makers or joining online communities for guidance on safety best practices.
Programming Your BMS Solution
Core Monitoring Functions
A robust BMS implementation on Raspberry Pi requires accurate monitoring of three critical parameters: voltage, current, and temperature. For voltage monitoring, connect the battery cells through a voltage divider circuit to the Pi’s ADC (Analog-to-Digital Converter) inputs. Since the Raspberry Pi doesn’t have built-in ADC capabilities, you’ll need to use an external ADC module like the ADS1115, which communicates via I2C protocol.
Current monitoring is achieved using a hall-effect sensor or shunt resistor connected to the ADC. The INA219 current sensor module is particularly suitable for this purpose, offering high precision measurements up to ±3.2A. For higher current applications, consider using the ACS712 sensor, which can handle up to 30A.
Temperature monitoring involves placing thermistors at strategic points on your battery pack. These can be read using a simple voltage divider circuit connected to the ADC, or you can opt for digital temperature sensors like the DS18B20, which connect directly to the Pi’s GPIO pins and provide accurate readings with minimal setup.
To implement these monitoring functions, use Python libraries such as Adafruit_ADS1x15 for the ADC readings and w1thermsensor for temperature sensors. Sample the readings at regular intervals (typically every 1-2 seconds) and store them in a database for tracking and analysis. This data forms the foundation for implementing protection features and optimization algorithms in your BMS.
Data Logging System
A robust data logging system is essential for monitoring and analyzing your BMS performance over time. Using the Raspberry Pi’s capabilities, you can set up a comprehensive logging solution that captures vital battery parameters and stores them for future analysis.
Start by creating a Python script that reads data from your BMS sensors at regular intervals. You can use popular libraries like pandas for data organization and SQLite for storage. Here’s a basic implementation approach:
First, install required dependencies through pip:
“`
pip install pandas sqlite3
“`
Configure your script to collect key metrics including voltage levels, current draw, temperature readings, and state of charge. Set up automated logging at specific intervals (typically every 5-15 minutes) to maintain a balanced approach between data granularity and storage efficiency.
For visualization and analysis, consider implementing Grafana or Matplotlib to create real-time dashboards. These tools can help you identify patterns, track battery health, and detect potential issues before they become critical.
To prevent storage issues, implement a rolling log system that maintains data for a specified period (e.g., 30 days) and automatically archives older entries. Consider setting up automatic backups to a network drive or cloud storage for data redundancy.
Remember to include error handling and logging mechanisms to capture any system anomalies or sensor failures. This helps in troubleshooting and maintaining system reliability over time.
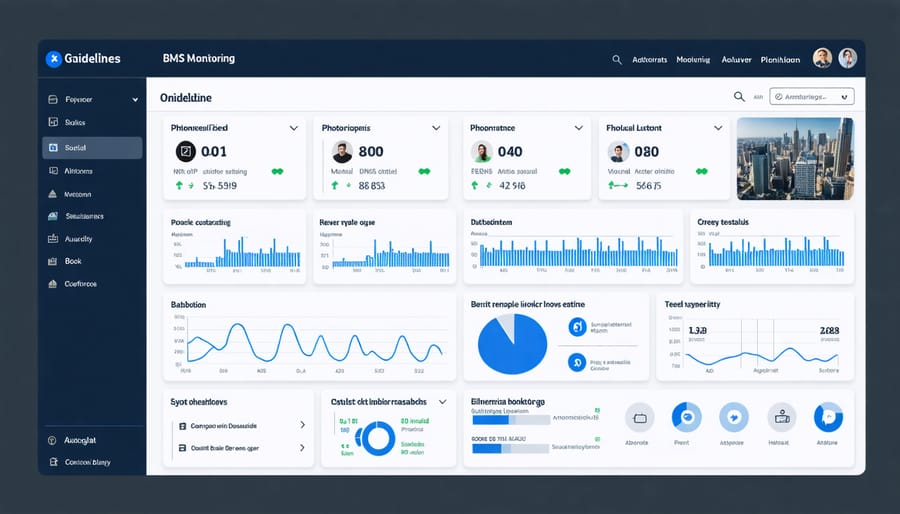
Alert System Configuration
A robust alert system is crucial for any BMS implementation on a Raspberry Pi to prevent battery damage and ensure safe operation. The system should monitor critical parameters and respond appropriately when thresholds are exceeded.
Start by implementing voltage monitoring alerts. Configure your system to trigger warnings when cell voltages approach their upper or lower limits. Set up three threshold levels: warning (approaching limits), critical (immediate action needed), and emergency shutdown. For Li-ion batteries, typical warning thresholds might be 3.0V (low) and 4.1V (high), with critical levels at 2.8V and 4.2V respectively.
Temperature monitoring is equally important. Configure alerts for both individual cell temperatures and overall battery pack temperature. Program your Raspberry Pi to initiate automatic shutdown procedures if temperatures exceed safe operating ranges (typically above 45°C or below 0°C).
Implement these alerts using Python’s GPIO pins to control visual indicators (LEDs) and audible alarms (buzzers). For remote monitoring, set up email notifications or push alerts to your smartphone using services like Pushbullet or IFTTT.
The emergency shutdown system should:
– Disconnect the main power relay
– Log the event with timestamp and sensor readings
– Send immediate notifications to designated contacts
– Maintain minimal system operation for diagnostic purposes
Remember to include fail-safes that trigger shutdown even if the main processor becomes unresponsive, using hardware-level protection circuits as backup.
Testing and Optimization
System Validation
After assembling your BMS system with Raspberry Pi, thorough validation is crucial for ensuring safe and reliable operation. Begin testing with a simple voltage measurement check across all battery cells. Use a calibrated multimeter to verify that the voltage readings from your BMS match the actual measurements within an acceptable margin of error (typically ±0.1V).
Next, test the temperature monitoring system by comparing readings from your temperature sensors with a known reference thermometer. Verify that the system responds correctly to temperature changes and triggers appropriate safety measures when thresholds are exceeded.
For troubleshooting common issues, first check all physical connections, ensuring proper wiring and secure GPIO connections. If you encounter communication errors, verify your I2C or SPI configuration in raspi-config. Temperature sensor failures often stem from incorrect pull-up resistor configurations or damaged sensor wiring.
Monitor your system’s data logging capability by running it for 24 hours and analyzing the recorded data for any anomalies or unexpected patterns. This extended testing period helps identify potential long-term stability issues before deploying the system in a production environment.
Performance Improvements
To enhance your BMS Raspberry Pi system’s performance, implementing several optimization techniques can make a significant difference. First, consider ways to optimize power consumption by utilizing sleep modes and efficient coding practices. Enable hardware PWM for more precise battery charging control and implement multi-threading to handle simultaneous monitoring tasks without overwhelming the processor.
Consider using a real-time database for logging battery data, which can significantly reduce storage overhead and improve read/write speeds. Implement buffer management for sensor readings to prevent data loss during high-frequency measurements. For advanced users, enabling GPU acceleration for complex calculations can drastically improve processing speed.
Memory management is crucial – use efficient data structures and implement cleanup routines to prevent memory leaks. Configure your system to use RAM-based temporary storage for frequently accessed data, reducing SD card wear and improving response times. Additionally, implementing watchdog timers can help maintain system stability by automatically recovering from potential crashes or hangs.
Finally, optimize your networking stack if you’re implementing remote monitoring capabilities, using efficient protocols like MQTT for data transmission.
Building a Battery Management System with a Raspberry Pi opens up exciting possibilities for DIY energy monitoring and storage solutions. Throughout this guide, we’ve explored the essential components, setup procedures, and programming requirements to create a functional BMS. By following these steps, you can monitor battery voltage, current, temperature, and implement safety features to protect your battery systems.
Remember to prioritize safety in your implementation, regularly test your system, and keep your code updated with the latest security patches. Whether you’re using this setup for a solar power system, electric vehicle project, or other applications, the flexibility of the Raspberry Pi makes it an excellent choice for custom BMS solutions.
Consider expanding your project by adding remote monitoring capabilities, implementing more advanced charging algorithms, or connecting multiple battery packs. The possibilities are endless, and with the foundation provided in this guide, you’re well-equipped to start your BMS journey.